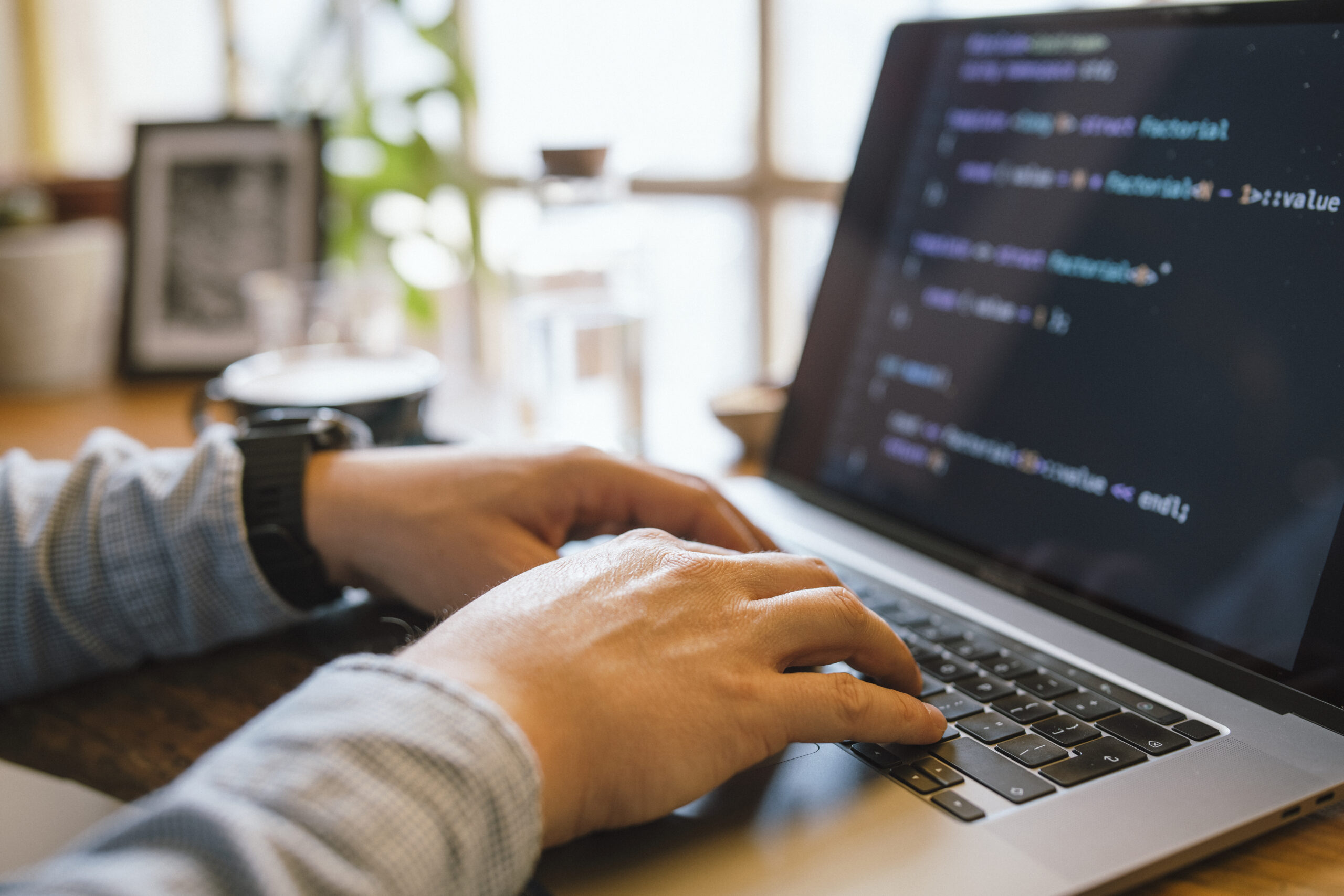
Debugging is Probably the most necessary — yet frequently neglected — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to Believe methodically to solve issues effectively. No matter whether you're a novice or even a seasoned developer, sharpening your debugging expertise can preserve hrs of disappointment and drastically transform your efficiency. Here i will discuss quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is one particular A part of development, recognizing tips on how to communicate with it successfully during execution is Similarly significant. Modern day progress environments arrive Geared up with highly effective debugging capabilities — but many builders only scratch the surface of what these applications can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and perhaps modify code within the fly. When used accurately, they Allow you to observe accurately how your code behaves in the course of execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-end builders. They allow you to inspect the DOM, monitor community requests, check out authentic-time efficiency metrics, and debug JavaScript while in the browser. Mastering the console, sources, and network tabs can convert frustrating UI troubles into workable duties.
For backend or process-level builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory management. Understanding these instruments can have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Variation control methods like Git to grasp code record, discover the exact second bugs have been launched, and isolate problematic variations.
Ultimately, mastering your resources signifies likely beyond default settings and shortcuts — it’s about creating an intimate knowledge of your advancement surroundings to ensure when troubles come up, you’re not misplaced at nighttime. The higher you recognize your equipment, the greater time it is possible to shell out resolving the particular dilemma in lieu of fumbling by the method.
Reproduce the challenge
One of the more significant — and infrequently missed — steps in efficient debugging is reproducing the issue. Prior to jumping into the code or making guesses, builders will need to make a steady surroundings or scenario where the bug reliably seems. Without the need of reproducibility, repairing a bug gets a recreation of chance, often bringing about squandered time and fragile code adjustments.
The first step in reproducing a dilemma is collecting as much context as feasible. Check with queries like: What actions led to The problem? Which setting was it in — improvement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you may have, the less difficult it gets to be to isolate the precise ailments beneath which the bug occurs.
When you finally’ve collected more than enough data, try to recreate the challenge in your local natural environment. This could indicate inputting exactly the same facts, simulating comparable user interactions, or mimicking process states. If the issue seems intermittently, contemplate crafting automated checks that replicate the edge scenarios or state transitions included. These tests not simply aid expose the problem and also stop regressions in the future.
Often, the issue may very well be setting-particular — it'd occur only on sure running programs, browsers, or under distinct configurations. Making use of instruments like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms may be instrumental in replicating such bugs.
Reproducing the challenge isn’t just a phase — it’s a state of mind. It involves persistence, observation, and also a methodical solution. But after you can continuously recreate the bug, you're presently midway to repairing it. That has a reproducible state of affairs, You need to use your debugging tools a lot more correctly, exam possible fixes securely, and converse extra Evidently with your team or customers. It turns an abstract complaint into a concrete obstacle — and that’s the place builders prosper.
Browse and Comprehend the Error Messages
Error messages tend to be the most precious clues a developer has when one thing goes Mistaken. In lieu of seeing them as irritating interruptions, builders should learn to treat mistake messages as immediate communications within the procedure. They normally inform you just what exactly occurred, exactly where it happened, and sometimes even why it transpired — if you know the way to interpret them.
Start out by examining the information thoroughly As well as in total. Numerous builders, particularly when below time pressure, look at the initial line and instantly start building assumptions. But deeper in the mistake stack or logs may possibly lie the correct root cause. Don’t just duplicate and paste mistake messages into engines like google — study and understand them 1st.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or a logic mistake? Will it place to a particular file and line quantity? What module or functionality activated it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also handy to grasp the terminology from the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally stick to predictable styles, and Understanding to acknowledge these can significantly hasten your debugging procedure.
Some glitches are vague or generic, and in Those people circumstances, it’s very important to examine the context through which the mistake occurred. Verify relevant log entries, input values, and up to date changes inside the codebase.
Don’t overlook compiler or linter warnings either. These generally precede larger troubles and supply hints about potential bugs.
Eventually, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, encouraging you pinpoint problems more quickly, lower debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is One of the more potent instruments inside of a developer’s debugging toolkit. When utilized properly, it offers authentic-time insights into how an software behaves, helping you comprehend what’s happening under the hood without needing to pause execution or stage with the code line by line.
A superb logging tactic starts off with figuring out what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic information during development, Facts for normal gatherings (like prosperous start out-ups), WARN for prospective problems that don’t break the applying, ERROR for real issues, and Lethal in the event the technique can’t proceed.
Stay away from flooding your logs with extreme or irrelevant data. Too much logging can obscure vital messages and decelerate your program. Give attention to crucial activities, state modifications, input/output values, and significant selection details as part of your code.
Format your log messages Evidently and persistently. Incorporate context, including timestamps, ask for IDs, and function names, so it’s simpler to trace concerns in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what ailments are met, and what branches of logic are executed—all without the need of halting the program. They’re especially worthwhile in manufacturing environments wherever stepping via code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, intelligent logging is about stability and clarity. Which has a properly-assumed-out logging strategy, you may reduce the time it requires to identify issues, obtain further visibility into your applications, and improve the Total maintainability and reliability of the code.
Assume Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To proficiently determine and correct bugs, builders must strategy the method similar to a detective resolving a mystery. This frame of mind allows break down sophisticated difficulties into workable parts and stick to clues logically to uncover the foundation cause.
Begin by gathering evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, gather as much related info as you'll be able to without having jumping to conclusions. Use logs, examination circumstances, and consumer reviews to piece with each other a clear picture of what’s going on.
Future, variety hypotheses. Talk to you: What can be resulting in this habits? Have any improvements not long ago been designed on the codebase? Has this situation transpired in advance of underneath equivalent conditions? The aim is always to narrow down choices and identify potential culprits.
Then, exam your theories systematically. Try and recreate the challenge in the controlled atmosphere. If you suspect a selected purpose or element, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the results direct you closer to the reality.
Spend shut focus to small facts. Bugs usually disguise from the least predicted locations—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and individual, resisting the urge to patch the issue without the need of completely comprehending it. Momentary fixes may possibly hide the true trouble, only for it to resurface afterwards.
And finally, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can help save time for long term difficulties and help Some others comprehend your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution difficulties methodically, and become simpler at uncovering concealed issues in intricate units.
Create Assessments
Crafting tests is one of the best solutions to improve your debugging abilities and Total progress performance. Tests not simply help catch bugs early but in addition function a security Web that offers you assurance when making adjustments in your codebase. A properly-examined software is much easier to debug mainly because it allows you to pinpoint specifically the place and when a challenge happens.
Begin with device assessments, which center on unique functions or modules. These smaller, isolated checks can immediately expose whether a specific piece of logic is working as envisioned. Any time a test fails, you immediately know where to glimpse, noticeably lessening some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear just after Earlier currently being mounted.
Subsequent, combine integration assessments and stop-to-finish tests into your workflow. These assistance be sure that a variety of areas of your software get the job done collectively easily. They’re especially practical for catching bugs that come about in intricate techniques with numerous factors or companies interacting. If one thing breaks, your checks can inform you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing exams also forces you to definitely Feel critically regarding your code. To test a feature adequately, you will need to know its inputs, envisioned outputs, and edge scenarios. This degree of being familiar with By natural means leads Gustavo Woltmann AI to higher code structure and less bugs.
When debugging an issue, composing a failing test that reproduces the bug is usually a powerful starting point. After the exam fails persistently, you can center on fixing the bug and observe your exam pass when The problem is fixed. This method makes sure that a similar bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a disheartening guessing sport into a structured and predictable approach—helping you catch far more bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the challenge—observing your monitor for several hours, seeking Answer right after Resolution. But One of the more underrated debugging applications is simply stepping away. Taking breaks assists you reset your thoughts, decrease disappointment, and sometimes see The problem from a new viewpoint.
When you're too near to the code for way too very long, cognitive exhaustion sets in. You may commence overlooking clear problems or misreading code you wrote just hrs previously. On this state, your brain becomes less efficient at trouble-resolving. A brief stroll, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also enable avert burnout, Specifically throughout longer debugging classes. Sitting before a display, mentally stuck, is not simply unproductive but additionally draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may well come to feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In short, getting breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your standpoint, and aids you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you come upon is more than just A brief setback—It can be a possibility to develop like a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, every one can instruct you something beneficial in case you make the effort to replicate and review what went Improper.
Start off by inquiring on your own some vital questions once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code evaluations, or logging? The answers usually reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding routines moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or retain a log where you Be aware down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically powerful. Irrespective of whether it’s by way of a Slack concept, a brief produce-up, or a quick awareness-sharing session, serving to Other folks avoid the exact situation boosts group performance and cultivates a more powerful Studying lifestyle.
A lot more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your advancement journey. After all, many of the very best builders aren't those who write best code, but those who repeatedly learn from their problems.
Eventually, Each and every bug you take care of adds a whole new layer to your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.